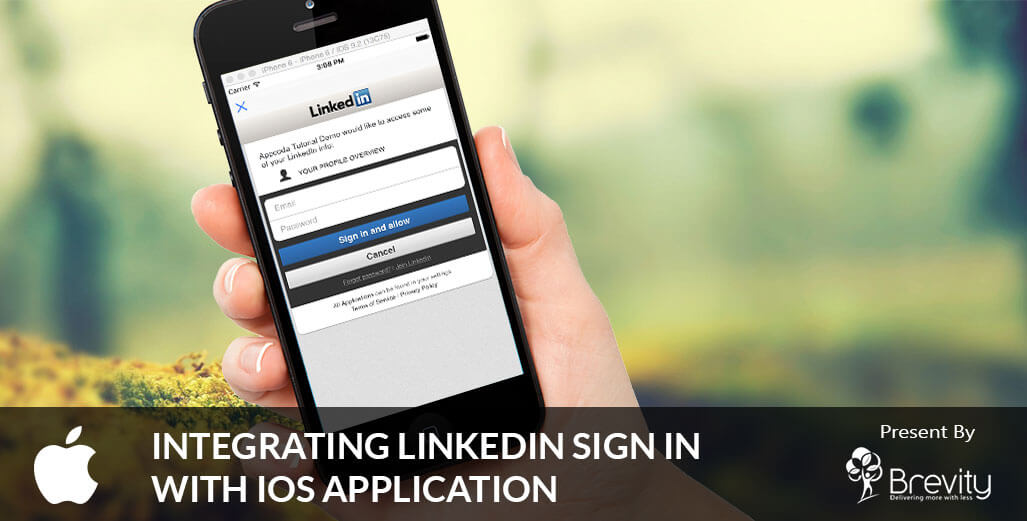
Let’s become proficient in how to integrate LinkedIn in your iOS application.
Note: This blog is about how to integrate LinkedIn Login in your iOS app with LinkedIn REST API. You can also integrate with LinkedIn Mobile SDK for that check the link below.
https://developer.linkedin.com/docs/signin-with-linkedin
Let’s start by creating New App in LinkedIn Developer
Step:1 Create New App in LinkedIn Developer
Login to LinkedIn Developer (Here is the link https://developer.linkedin.com/)
Select MyApps -> Create Application
Fill in all the details to create a new application. All fields are mandatory.
After filling in all the detail click on submit button.
You can find ClienID and ClientSecret from there. Also, you can update application permissions and Authorized Redirect URLs.
r_basicprofile is by default checked, if you want to fetch email then also check r_emailaddress.
Then we have to provide Authorized Redirect URL inside OAuth 2.0 section. It is needed when a user tries to refresh an existing access token. The user does not require to log in again if he has already login previously, the OAuth flow will automatically redirect the app using that URL. For the normal login process that URL is exchanged between the client App and the server while fetching the Authorization code and access token.
The authorization URL should not real or existing URL. You can provide any value starting with “https://”.
Don’t forget to click the update button after changing all information.
Step:2 Create XCode project name it “LILoginDemo”.
Step:3 Create UI For Application
In your viewController add labels for firstName, lastName, email, Id, public url, if you want to display other details then also add labels for them.
Add 2 buttons Login Using LinkedIn and Get Profile Details.
Add another view controller and add webview inside it.
Add a WebViewController.swift file assign it to another view controller in the storyboard.
Give segue from Login Using LinkedIn button to another view controller.
Step:4 Prepare Request to get Authorization Code
In your WebViewController class add constants for linkedInKey, linkedInSecret, authorizationEndPoint and accessTokenEndPoint.
Assign value of Client Id to linkedInKey and value of Client Secret to linkedInSecret.
Assign https://www.linkedin.com/uas/oauth2/authorization to authorizationEndPoint which is used for requests.
Assign https://www.linkedin.com/uas/oauth2/accessToken to accessTokenEndPoint which is used to get accessToken.
let linkedInKey = "759w69ead3dlej"
let linkedInSecret = "7Fw3tWZTGEhAnKAY"
let authorizationEndPoint = "https://www.linkedin.com/uas/oauth2/authorization"
let accessTokenEndPoint = "https://www.linkedin.com/uas/oauth2/accessToken"
Our main goal in this step is to prepare the request for getting the authorization code and to load it through a web view.
The request to get an authorization code must require the following parameters:
response_type: It’s a standard value that must always be: code
Client_id: It’s the Client ID value taken from the LinkedIn Developer’s website and been assigned to the linkedInKey
redirect_uri: The authorized redirection URL value that you have specified while creating an application in LinkedIn Developer’s website.
state: A unique string is required to prevent a cross-site request forgery (CSRF).
scope: A URL-encoded list of the permissions that our app requests.
Now create a method in WebViewController with name startAuthorization().
Inside this method specify request parameters as shown in below code snippet.
// Specify the response type which should always be "code".
let responseType = "code"
// Set the redirect URL which you have specify at time of creating application in LinkedIn Developer’s website. Adding the percent escape characthers is necessary.
let redirectURL = “https://com.test.linkedin/oauth”.stringByAddingPercentEncodingWithAllowedCharacters(NSCharacterSet.alphanumericCharacterSet())!
// Create a random string based on the time interval (it will be in the form linkedin12345679).
let state = “linkedin\(Int(NSDate().timeIntervalSince1970))”
// Set preferred scope.
let scope = “r_basicprofile, r_emailaddress”
The next step is to compose the authorization URL. For that use URL assigned to authorizationEndPoint, and append all parameters.
// Create the authorization URL string.
var authorizationURL = "\(authorizationEndPoint)?"
authorizationURL += "response_type=\(responseType)&"
authorizationURL += "client_id=\(linkedInKey)&"
authorizationURL += "redirect_uri=\(redirectURL)&"
authorizationURL += "state=\(state)&"
authorizationURL += "scope=\(scope)"
print(authorizationURL)
Finally, we have to load the request in webview. Make sure that you have passed the correct parameters otherwise you are not able to log in.
To load a request in webview you just need to write a couple of lines in startAuthorization() method.
// Create a URL request and load it in the web view.
let request = NSURLRequest(URL: NSURL(string: authorizationURL)!)
webView.loadRequest(request)
Call startAuthorization() from viewDidLoad().
We have to check in webview delegate method that the request URL is the one we are looking for and also we must ensure that the authorization code exists in the LinkedIn response. Then get authorization code by separating string and call requestForAccessToken(authorizationCode: String) method and pass authorization code as parameter.
Step:5 Request for Access Token
Inside requestForAccessToken(authorizationCode: String) method, request for access token using NSMutableURLRequest.
Let’s prepare post parameters for access token request
grant_type: It’s a standard value that should always be: authorization_code
code: The authorization code acquired in the previous part.
redirect_uri: It’s the authorized redirection URL we’ve talked about many times earlier.
client_id: The Client Key value.
client_secret: The Client Secret Value.
Specify grant type and redirect url and set all POST parameters.
let redirectURL = "https://com.appcoda.linkedin.oauth/oauth".stringByAddingPercentEncodingWithAllowedCharacters(NSCharacterSet.alphanumericCharacterSet())!
// Set the POST parameters.
var postParams = "grant_type=\(grantType)&"
postParams += "code=\(authorizationCode)&"
postParams += "redirect_uri=\(redirectURL)&"
postParams += "client_id=\(linkedInKey)&"
postParams += "client_secret=\(linkedInSecret)"
Set parameters to request body, set HTTPMethod to POST, set content type = application/x-www-form-urlencoded to HTTPHeaderField, After all setting send request.
You will receive access token in response, store it in NSUserDefault and dismiss view controller.
Full code snippet for requestForAccessToken(authorizationCode: String) method given below.
func requestForAccessToken(authorizationCode: String) {
let grantType = "authorization_code"
let redirectURL = “https://com.test.LinkedIn/oauth”.stringByAddingPercentEncodingWithAllowedCharacters(NSCharacterSet.alphanumericCharacterSet())!
// Set the POST parameters.
var postParams = “grant_type=\(grantType)&”
postParams += “code=\(authorizationCode)&”
postParams += “redirect_uri=\(redirectURL)&”
postParams += “client_id=\(linkedInKey)&”
postParams += “client_secret=\(linkedInSecret)”
// Convert the POST parameters into a NSData object.
let postData = postParams.dataUsingEncoding(NSUTF8StringEncoding)
// Initialize a mutable URL request object using the access token endpoint URL string.
let request = NSMutableURLRequest(URL: NSURL(string: accessTokenEndPoint)!)
// Indicate that we’re about to make a POST request.
request.HTTPMethod = “POST”
// Set the HTTP body using the postData object created above.
request.HTTPBody = postData
// Add the required HTTP header field.
request.addValue(“application/x-www-form-urlencoded;”, forHTTPHeaderField: “Content-Type”)
// Initialize a NSURLSession object.
let session = NSURLSession(configuration: NSURLSessionConfiguration.defaultSessionConfiguration())
// Make the request.
let task: NSURLSessionDataTask = session.dataTaskWithRequest(request) { (data, response, error) -> Void in
// Get the HTTP status code of the request.
let statusCode = (response as! NSHTTPURLResponse).statusCode
if statusCode == 200 {
// Convert the received JSON data into a dictionary.
do {
let dataDictionary = try NSJSONSerialization.JSONObjectWithData(data!, options: NSJSONReadingOptions.MutableContainers)
let accessToken = dataDictionary[“access_token”] as! String
NSUserDefaults.standardUserDefaults().setObject(accessToken, forKey: “LIAccessToken”)
NSUserDefaults.standardUserDefaults().synchronize()
dispatch_async(dispatch_get_main_queue(), { () -> Void in
self.dismissViewControllerAnimated(true, completion: nil)
})
}
catch {
print(“Could not convert JSON data into a dictionary.”)
}
}
}
task.resume()
}
Step:6 Check for Existance of Access Token
Now in your ViewController.swift file check for Existance of the access token and if it already exists then no need to ask the user to log in, so disable Login with LinkedIn Button and enable Get Profile Button.
func checkForExistingAccessToken() {
if NSUserDefaults.standardUserDefaults().objectForKey("LIAccessToken") != nil {
btnSignIn.enabled = false
btnGetProfileInfo.enabled = true
}
}
Call checkForExistingAccessToken() method from viewWillAppear()
Step:7 Fetching the user profile
On getting the Profile Details button tap request for user profile detail.
For that you we have to make a GET request to the LinkedIn server by using an access token asking for the user’s profile detail.
You have to pass required parameters name within URL inside bracket for example,
https://api.linkedin.com/v1/people/~:(id,public-profile-url,first-name,last-name,email-address)?format=json
Here id, public-profile-url, first-name, last-name, email-address are parameters name. If you want more detail then you can pass respecting parameter. You can find a list of parameters here (https://developer.linkedin.com/docs/fields/basic-profile).
After getting success in REST API response then get all detail from the dictionary and display it in the specific label.
Full code snippet for getProfileInfo method is given below:
@IBAction func getProfileInfo(sender: AnyObject) {
if let accessToken = NSUserDefaults.standardUserDefaults().objectForKey("LIAccessToken") {
// Specify the URL string that we'll get the profile info from.
let targetURLString = "https://api.linkedin.com/v1/people/~:(id,public-profile-url,first-name,last-name,email-address)?format=json"
// Initialize a mutable URL request object.
let request = NSMutableURLRequest(URL: NSURL(string: targetURLString)!)
// Indicate that this is a GET request.
request.HTTPMethod = “GET”
// Add the access token as an HTTP header field.
request.addValue(“Bearer \(accessToken)”, forHTTPHeaderField: “Authorization”)
// Initialize a NSURLSession object.
let session = NSURLSession(configuration: NSURLSessionConfiguration.defaultSessionConfiguration())
// Make the request.
let task: NSURLSessionDataTask = session.dataTaskWithRequest(request) { (data, response, error) -> Void in
// Get the HTTP status code of the request.
let statusCode = (response as! NSHTTPURLResponse).statusCode
if statusCode == 200 {
// Convert the received JSON data into a dictionary.
do {
let dataDictionary = try NSJSONSerialization.JSONObjectWithData(data!, options: NSJSONReadingOptions.MutableContainers)
print(“response dictionary ::: \(dataDictionary)”)
dispatch_async(dispatch_get_main_queue(), { () -> Void in
if let profileURLString = dataDictionary[“publicProfileUrl”] {
self.lblPublicUrl.text = profileURLString as? String
}
if let firstName = dataDictionary[“firstName”] {
self.lblFirstName.text = firstName as? String
}
if let lastName = dataDictionary[“lastName”] {
self.lblLastName.text = lastName as? String
}
if let userId = dataDictionary[“id”] {
self.lblId.text = userId as? String
}
if let email = dataDictionary[“emailAddress”] {
self.lblEmail.text = email as? String
}
})
}
catch {
print(“Could not convert JSON data into a dictionary.”)
}
}
}
task.resume()
}
}
Summary:
All you have to do for LinkedIn Login and fetch user profile
- Create application in LinkedIn Developer.
- Set authorization redirect URL and default application permission.
- Prepare request to get authorization code and load it in webview.
- Get Access Token using authorization code and store it in user default.
- Using Access token get user profile info.